DRV8805 Chip Testing
Hardware wise the project is close to completion. The MUP Astro hat now has an enclosure that exposes the PI’s USB sockets with some rubber sealing around the edges/in-between. A 6p6c connector for the focuser, a short serial cable for the autostar handset, dual power input lines via a 6 pin connector and 3x2.5mm output sockets to power the main CCD camera (2.5mm->2.1mm cable), LX90 and dew heater.
The Astro Hat, CCD and LX90 all share the same 12v power line “A” whilst the dew heater has exclusive use of line “B” to limit noise due to its use of PWM.
Before writing a libindi driver for the focuser and temperature hardware I wanted to first test the hardware on its own. For testing the focuser DRV8805 chip I made use of the gpio command line app which provides a way to easily control the PIs GPIO pins.
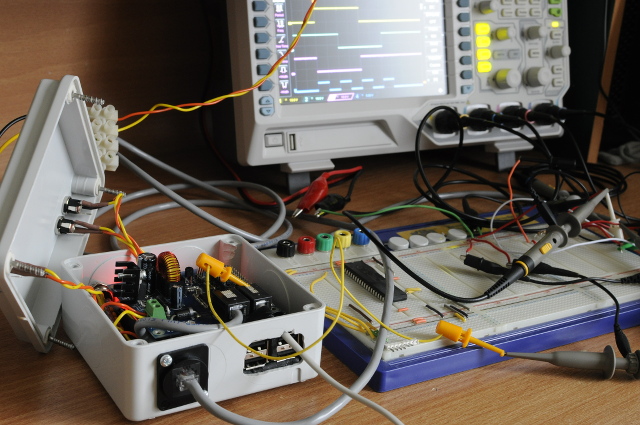
MUP Astro Hat within final enclosure
Test Scripts
The DRV8805 removes the timing burden from the PI allowing the motor to be driven a step at a time by toggling the STEP pin. Three stepping modes are supported, Full, Half and Wave. Below is a script to setup the pins for full stepping, although I made sure to test the output of all three modes in both clockwise and counter-clockwise directions.
Running
gpio readall
+-----+-----+---------+------+---+---Pi 3---+---+------+---------+-----+-----+
| BCM | wPi | Name | Mode | V | Physical | V | Mode | Name | wPi | BCM |
+-----+-----+---------+------+---+----++----+---+------+---------+-----+-----+
| | | 3.3v | | | 1 || 2 | | | 5v | | |
| 2 | 8 | SDA.1 | IN | 1 | 3 || 4 | | | 5V | | |
| 3 | 9 | SCL.1 | IN | 1 | 5 || 6 | | | 0v | | |
| 4 | 7 | GPIO. 7 | IN | 1 | 7 || 8 | 1 | ALT0 | TxD | 15 | 14 |
| | | 0v | | | 9 || 10 | 1 | ALT0 | RxD | 16 | 15 |
| 17 | 0 | GPIO. 0 | IN | 0 | 11 || 12 | 0 | IN | GPIO. 1 | 1 | 18 |
| 27 | 2 | GPIO. 2 | IN | 0 | 13 || 14 | | | 0v | | |
| 22 | 3 | GPIO. 3 | IN | 0 | 15 || 16 | 0 | IN | GPIO. 4 | 4 | 23 |
| | | 3.3v | | | 17 || 18 | 0 | IN | GPIO. 5 | 5 | 24 |
| 10 | 12 | MOSI | IN | 0 | 19 || 20 | | | 0v | | |
| 9 | 13 | MISO | IN | 0 | 21 || 22 | 0 | IN | GPIO. 6 | 6 | 25 |
| 11 | 14 | SCLK | IN | 0 | 23 || 24 | 1 | IN | CE0 | 10 | 8 |
| | | 0v | | | 25 || 26 | 1 | IN | CE1 | 11 | 7 |
| 0 | 30 | SDA.0 | IN | 1 | 27 || 28 | 1 | IN | SCL.0 | 31 | 1 |
| 5 | 21 | GPIO.21 | IN | 1 | 29 || 30 | | | 0v | | |
| 6 | 22 | GPIO.22 | IN | 1 | 31 || 32 | 0 | IN | GPIO.26 | 26 | 12 |
| 13 | 23 | GPIO.23 | OUT | 0 | 33 || 34 | | | 0v | | |
| 19 | 24 | GPIO.24 | OUT | 0 | 35 || 36 | 0 | OUT | GPIO.27 | 27 | 16 |
| 26 | 25 | GPIO.25 | OUT | 0 | 37 || 38 | 0 | OUT | GPIO.28 | 28 | 20 |
| | | 0v | | | 39 || 40 | 0 | OUT | GPIO.29 | 29 | 21 |
+-----+-----+---------+------+---+----++----+---+------+---------+-----+-----+
| BCM | wPi | Name | Mode | V | Physical | V | Mode | Name | wPi | BCM |
+-----+-----+---------+------+---+---Pi 3---+---+------+---------+-----+-----+
provides a listing of the physical header pin numbers along with the corresponding BCM and GPIO (wiring pi) pin numbers needed by the gpio util and the current INPUT/OUTPUT state and value of the pin.
The following script sets the relevant pins to OUTPUTs and writes the values needed for Full step mode in the counter-clockwise direction.
#!/bin/sh
# header pin num.
nENABLE=29
RESET=28
SM1=25
SM0=27
DIR=24
STEP=23
gpio mode ${nENABLE} out
gpio mode ${RESET} out
gpio mode ${SM1} out
gpio mode ${SM0} out
gpio mode ${DIR} out
gpio mode ${STEP} out
# Enter Reset
gpio write ${RESET} 1
# Set enabled, full step and CCW dir
gpio write ${nENABLE} 0
gpio write ${SM1} 0
gpio write ${SM0} 0
gpio write ${DIR} 0
gpio write ${STEP} 0
# Exit reset
gpio write ${nENABLE} 0
gpio readall
Three of the oscilloscope probes were hooked up to the driver chip outputs. I can only monitor three of the four outputs at once as the 4th channel on the oscilloscope was needed as a trigger via the STEP pin.
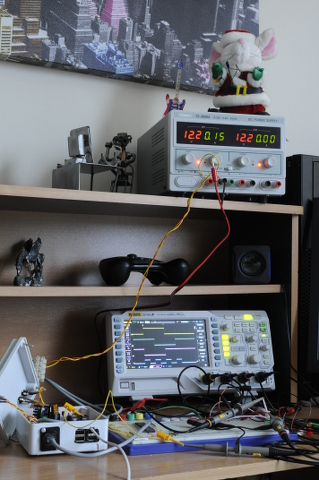
MUP Astro Hat performing slow stepping of the DRV8805
The unipolar motor will have four coil windings with 12v applied between them as shown in the wiring diagram on the beckwithelectronics page. The DRV8805 grounds the four outputs in a specific sequence to allow the motor to spin. As the focuser has not yet arrived, I made use of four resistors (right side of breadboard) between 12v and the driver chip output pins as a rough stand-in for the resistive windings.
At this point, stepping the “motor” is trivial. Drive the STEP pin high for at least 1.9uS then low again (can optionally be kept high to keep the windings energised).
#!/bin/sh
# header pin num.
STEP=23
nHOME=26
echo Stepping once
gpio write ${STEP} 1
sleep 0.5
gpio write ${STEP} 0
echo /HOME is:
gpio read ${nHOME}
Output Signals
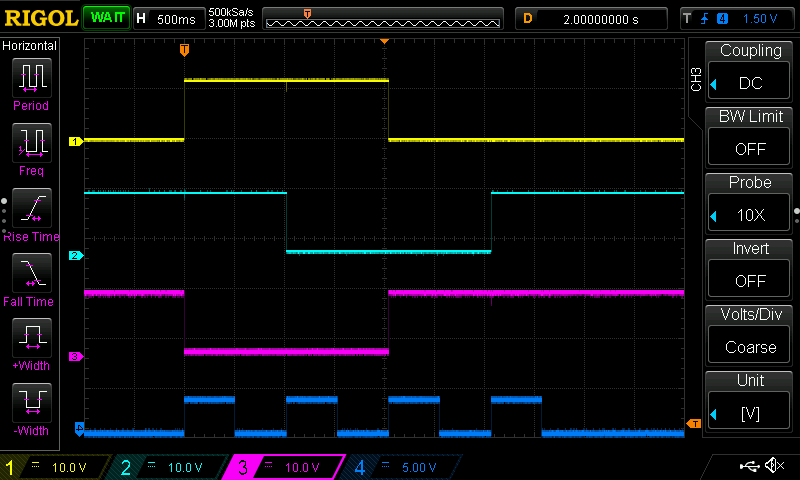
Out 1-3 Full Step sequence
The above shows four channels. Top to bottom they’re out1 (yellow), out2 (cyan), out3 (purple) and the STEP pin (blue). The trace starts just after the setup script has run and the chip has just been reset. out1 low, out2 high, out3 high. Note, low means that “coil” is On whilst a high is off due to the 12v common.
Four steps are needed to complete a full cycle from /HOME state back to /HOME again when using full stepping. As the scope trace shows, the bottom blue signal has four steps of 0.5s each with a 0.5s gap between the steps. The out1..3 traces switch on the rising edge of the STEP signal.
Step # | Out1 | Out 2 | Out 3 | Out 4 |
---|---|---|---|---|
Reset | On | Off | Off | On |
1 | Off | Off | On | On |
2 | Off | On | On | Off |
3 | On | On | Off | Off |
4 /HOME | On | Off | Off | On |
This matches the datasheet for a CCW dir full stepping cycle.
I did notice a quirk when testing half and wave stepping modes however. After a chip reset, in full step mode, the RESET state and /HOME states are the same and as expected it takes four steps to go from reset back to home and four more steps for each subsequent /HOME to /HOME cycle.
With half and wave mode after a reset it takes one extra step (9 for half step, 5 for wave) to go from the RESET state back to /HOME with the first step causing no change to the out signals. Continuing on from /HOME to /HOME and each subsequent cycle however takes the expected number of steps (8 for half step, 4 for wave).
A minor issue all told, but one that is not mentioned in the datasheet and could result in a off by one step directly after a reset if the /HOME is not also monitored for the initial 0->1 transition.
Open Hardware
With the driver chip working, that’s all chips now checked and confirmed working. I’ve fixed the design flaws noted in prior posts and updated the schematic/pcb/gerber files accordingly.
These are now available on github at:-
- https://github.com/Sector14/mup-astro-cat/
- https://github.com/Sector14/mup-astro-cat-hardware/
- https://github.com/Sector14/mup-kicad-library/
I’ve also tagged the current hardware as revision 2. Whilst this revision is a working version it still has the 100uF capacitor in a extended 1206 footprint requirement. That really needs changing to a footprint suitable for a cheaper electrolytic cap.
All that remains to do is hooking up a temperature sensor to the header pin then it’s onto software with a libindi driver needed for the focuser & temperature sensor and the eeprom overlay.
I’m looking forward to having the drivers working and being able to do a test with a complete scope/focuser/camera setup. At that time I’ll also be able to see how well it drives an actual stepper motor and how noisy the PSU may be under various loads and then it’ll finally be time to try it under the stars.